Homework 4 - ISBN Checker
(Credit to John Motil,
SIGCSE Nifty Assignments
2006.)
The
International Standard Book
Number (ISBN) is a unique identifier for books. The old standard, ISBN-10,
calls for 10 digits, laid out as follows (from
wikipedia):
- The group identifier (language)
- The publisher code
- The item number (title)
- A checksum or check digit
The check digit is calculated from the previous digits of the ISBN and is
used as a form of error detection. For example, if a digit is mistyped (e.g.,
by a distributor trying to specify which book they want 10,000 copies of), the
check digit catches that error. This is a simple version of an
error
detection code which are common in computer science. They are used in a
variety of applications to ensure that a piece of data has not been corrupted,
e.g., packets over the Internet, hard disk drives, and computer memory.
The check digit is calculated by first summing the first digit plus two
times the second digit plus third times the third all the way to nine times the
ninth digit. The remainder of this sum when divided by 11 is then computed.
If the remainder is 10, then the check symbol is "X". Otherwise, the check
symbol is the remainder. That is, the calculation is
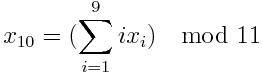
.
For example, consider the number 0205080057. This calculation on the first
nine digits yields 7 which is the 10th (check) digit of the number. Thus the
number is a valid ISBN.
The new standard, ISBN-13 is similar to a ISBN-10 except that the first
three digits designate the industry under which this product belongs. In the
case of books, these first three digits will always be 978.
However, because the format of the code is different, the calculation of the
check digit must also change. To the calculate the check digit of an ISBN-13
code, you sum the first 12 digits of the code like with ISBN-10. However, in
addition to summation, you also alternate multiplying each digit by 1 or 3, from
left to right, during the summation process. The result is taken modulo 10,
giving a value from 0 to 9 and that value is subtracted from 10, giving a final
result in the range 1 to 10. The check digit is 0 if the final result is 10.
Otherwise it is simply the final result itself. To summarize, the calculation
for ISBN-13 codes is
Your task is to write a program, ISBNChecker, that will prompt the user to the
type of ISBN code they wish to check, the code itself, and then report if that
code is valid. Your program should be in a class called
ISBNChecker in a
file called
ISBNChecker.java.
Output
Here are several executions of the program highlighting the different cases
that can occur. Input from the user is both
bolded and
underlined.
Sample execution #1:
This program checks to see if a given ISBN is valid.
It can process both ISBN-10 and ISBN-13 codes.
What kind of ISBN do you want to check? [ISBN-10, ISBN-13]: ISBN-10
Enter the ISBN-10 code to check: 0136091814
0136091814 is a valid ISBN-10 code.
Sample execution #2:
This program checks to see if a given ISBN is valid.
It can process both ISBN-10 and ISBN-13 codes.
What kind of ISBN do you want to check? [ISBN-10, ISBN-13]: ISBN-10
Enter the ISBN-10 code to check: 0136091812
0136091812 is not a valid ISBN-10 code.
Expected 4, but found 2.
Sample execution #3:
This program checks to see if a given ISBN is valid.
It can process both ISBN-10 and ISBN-13 codes.
What kind of ISBN do you want to check? [ISBN-10, ISBN-13]: ISBN-13
Enter the ISBN-13 code to check: 9780136091813
9780136091813 is a valid ISBN-13 code.
Sample execution #4:
This program checks to see if a given ISBN is valid.
It can process both ISBN-10 and ISBN-13 codes.
What kind of ISBN do you want to check? [ISBN-10, ISBN-13]: ISBN-13
Enter the ISBN-13 code to check: 9780136091817
9780136091817 is not a valid ISBN-13 code.
Expected 3, but found 7.
Sample execution #5:
This program checks to see if a given ISBN is valid.
It can process both ISBN-10 and ISBN-13 codes.
What kind of ISBN do you want to check? [ISBN-10, ISBN-13]: Cake
Cake is an invalid ISBN type.
Sample execution #6:
This program checks to see if a given ISBN is valid.
It can process both ISBN-10 and ISBN-13 codes.
What kind of ISBN do you want to check? [ISBN-10, ISBN-13]: ISBN-10
Enter the ISBN-10 code to check: 123456789X
123456789X is a valid ISBN-10 code.
(The above ISBN codes are from our textbook.)
As you can tell from the above examples, the program produces a
preamble that describes what the program does and then prompts the user
for type of ISBN to check and then the code. The program then computes the
check and then reports back to the user is the expected check digit matches the
actual check digit.
Your solution must reproduce the above output exactly. In particular,
format, capitalization, punctuation, and spacing should all be preserved. Note
when a user input is echoed in the output, e.g., "ISBN-13" and "Cake" in
execution #5.
When the user enters in the type of ISBN they want to check, they may enter
either "ISBN-10" or "ISBN-13". Your program should check for these strings
exactly, i.e., "isbn-10", "iSbn-13", and "ISBn-10" are all examples of invalid
types. When an invalid type is given, the program prints the above error
message and exits without computing anything.
In contrast, you can assume that the code given by the user is a string of
digits of the appropriate length. You do not need to check the length of the
input to ensure that it is valid or individual characters to ensure that they
are digits.
Design
Unlike previous homeworks, there is no visual pattern for you to decompose.
However, the principles of decomposition still apply! You should have at least
3 static methods that perform useful functionality and decompose the
problem of computing ISBNs in a meaningful way. As a first step, you should
look at the example runs above and split up the output based on functionality.
That division should suggest a beginning set of methods to write. There is
certainly room for more opportunities to decompose the problem further, so keep
an eye out for them!
Throughout this homework, you will need to convert from chars to ints and
vice versa. As we discussed in class, you must be wary because a char can
automatically converted into an int, but the resulting value is the
character
code of the char rather than the
numeric value of the char if it happens
to be a digit. This frequently results in confusion because this is an
error not caught by the compiler and (in Java, at least) can only be caught via
testing. Chapter 4.3 of the text explains these pitfalls and how to avoid them
in more detail.
Testing
Even though we give you a few sample executions for you to mimic, their
success does not guarantee that your program will run correctly! When we test
your program, we will try out other ISBNs to make sure that your program works
in a variety of cases.
Therefore, you should try out your program on other books that you own to
gain confidence that it indeed works on all inputs. Books published before 2007
will only have an ISBN-10 code, whereas post-2007 books typically feature both
an ISBN-10 and ISBN-13 code on the back.
Note that, in rare cases, ISBN codes may be entered incorrectly by the
publisher from the beginning which results in invalid ISBNs on books. In the
off-chance that your book's ISBN does not validate and you're sure that you've
done everything right, try another book.
Submission
Please
submit
your Java source file,
ISBNChecker.java, electronically via the
course website.